03.27.13
Posted in Uncategorized at 8:28 pm by danvk
My group recently launched a custom UI for March Madness searches:
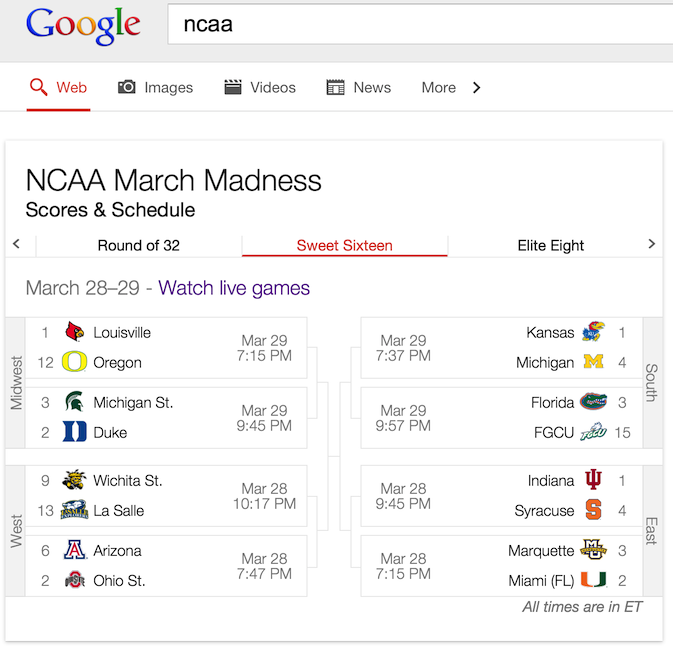
The Sweet Sixteen view looks particularly nice on tablets, where you get high resolution team logos and crisp text for the team names. While games are being played, you can follow the scores live in the bracket.
The launch was an interesting experience. I wrote a tweet shortly after we went live. It immediately got picked up by TechCrunch, Search Engine Land and Fred Wilson.
There are some slightly wild CSS tricks going on to mirror the bracket on the right-hand side and to make substitute team abbreviations when their full names won’t fit. Fodder for a future danvk.org post!
Permalink
03.03.13
Posted in Uncategorized at 11:25 am by danvk
I sometimes see code like this to generate DOM structures in JavaScript:
var div = document.createElement("div");
div.innerHTML = "<div id='foo' onclick='document.getElementById(\"foo\").style.display=\"none\";' style='position: absolute; top: 10px; left: 10px;'>" + content + "</div>";
document.body.appendChild(div);
This style gets very confusing very quickly. The issue is that it uses JavaScript to write HTML, CSS and even JavaScript inside HTML!
The key to untangling these knots is to remember this rule:
Keep your HTML in your HTML, your JavaScript in your JavaScript and your CSS in your CSS.
Here’s how I’d rewrite that snippet with this in mind:
HTML:
<div id='foo-template' class='foo' style='display:none;'>
</div>
CSS:
.foo {
position: absolute;
top: 10px;
left: 10px;
}
JavaScript:
var $foo = $('#foo-template').clone().removeAttr('id');
$foo
.on('click', function() { $(this).hide(); })
.text(content)
.appendTo(document.body)
.show();
The idea is that JavaScript is a really terrible language for building DOM structures. You can use either the innerHTML technique (in which case you run into quoting issues) or the DOM manipulation APIs (which are quite cumbersome and verbose).
HTML is a great way to define DOM structures! So define your DOM structures there, even the ones that you’ll add dynamically. You can use jQuery’s clone method to make copies of them that you fill out before adding them to the page.
CSS is also a great language for defining styles, so why put them inline in your HTML? Just use a class and move the styles into your CSS.
And really, do you want to write JavaScript that writes HTML that includes JavaScript?
You can see a real-world example of this technique in OldSF’s HTML, JS and CSS.
Permalink